Rectangle
Include <mln/core/se/rect2d.hpp>
-
struct rect2d : public mln::se_facade<rect2d>
Define a dynamic rectangular window anchored at (0,0). Its width and height are always odd numbers to ensure symmetry.
Public Functions
-
rect2d() = default
Construct an empty rectangle.
-
rect2d(int width, int height)
Construct a rectangle of size (Width × Height).
- Parameters:
width – The width of the rectangle. If
width
is even, it is rounded to the closest lower odd int.height – The height of the rectangle. If
height
is even, it is rounded to the closest lower odd int.
-
inline auto offsets() const
Return a range of SE offsets.
-
bool is_decomposable() const
Return true if decomposable (for any non-empty rectangle)
-
bool is_separable() const
Return true if separable (for any non-empty rectangle)
-
bool is_incremental() const
Return true if incremental (if the width is larger than 1)
-
std::vector<periodic_line2d> decompose() const
Return an horizontal line of length
Width
and a vertical line of lengthHeight
corresponding to the SE decomposition.
-
std::vector<periodic_line2d> separate() const
Return an horizontal line of length
Width
and a vertical line of lengthHeight
.
-
int radial_extent() const
Return the extent radius.
-
rect2d() = default
Performance
The rectangle is decomposable in two lines, so that the speed of a dilation (or erosion) by a rectangle does not depend on the size of the rectangle.
Example 1. Dilation by rectangle
#include <mln/core/se/rect2d.hpp>
mln::se::rect2d (21, 21);
auto output = mln::morpho::structural::dilate(input, rect);
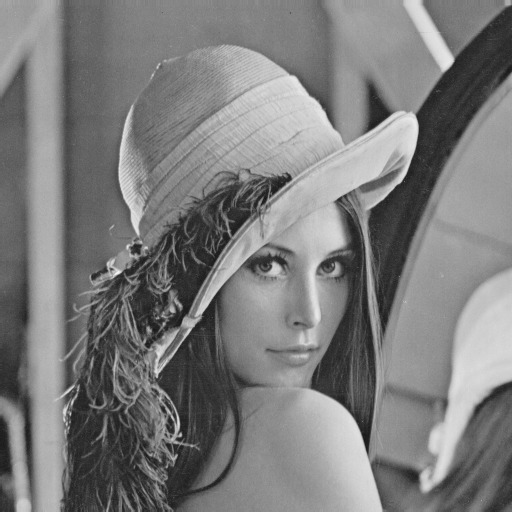
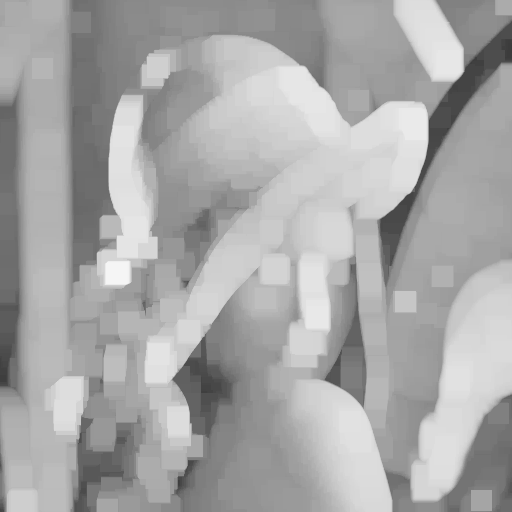